This article was originally written by Kevin Garcia and published in June 2021. It has been updated for 2024.
Maps might sound niche, but they’re hardly rare. React applications—and applications in general—often need maps. For some applications, like an app built to visualize inventory stock across store locations, a map might even be the bread and butter of the app’s value proposition. For other apps, like a company address display, maps might be more of a nice-to-have than a necessity, but a frequent element nonetheless.
But even the most basic maps are complicated, with spatial dimensions, dynamic zoom, labels, markers, color codes, and more—building a mapping feature from scratch is generally out of the question. Thankfully, React libraries can help developers readily address more complex mapping use cases.
These libraries fall into two categories. Some are tight wrappers around proprietary mapping data, such as the Google Maps API or Mapbox ecosystem. Others are libraries that convert input map data (such as OpenStreetMap) into visual displays. Some libraries favor simplicity and performance; others prioritize feature coverage.
This post breaks down four major mapping libraries, each with its own use case, to help you identify which React mapping library is best for you. These libraries have strong adoption, with none dipping below 50,000 weekly downloads. Let’s dig into each of their design philosophies, and how they can help you reach your specific project goals.
The omnipresent, Canvas-powered favorite
GitHub Stars: 6.3k
NPM weekly downloads: 230k
License: MIT
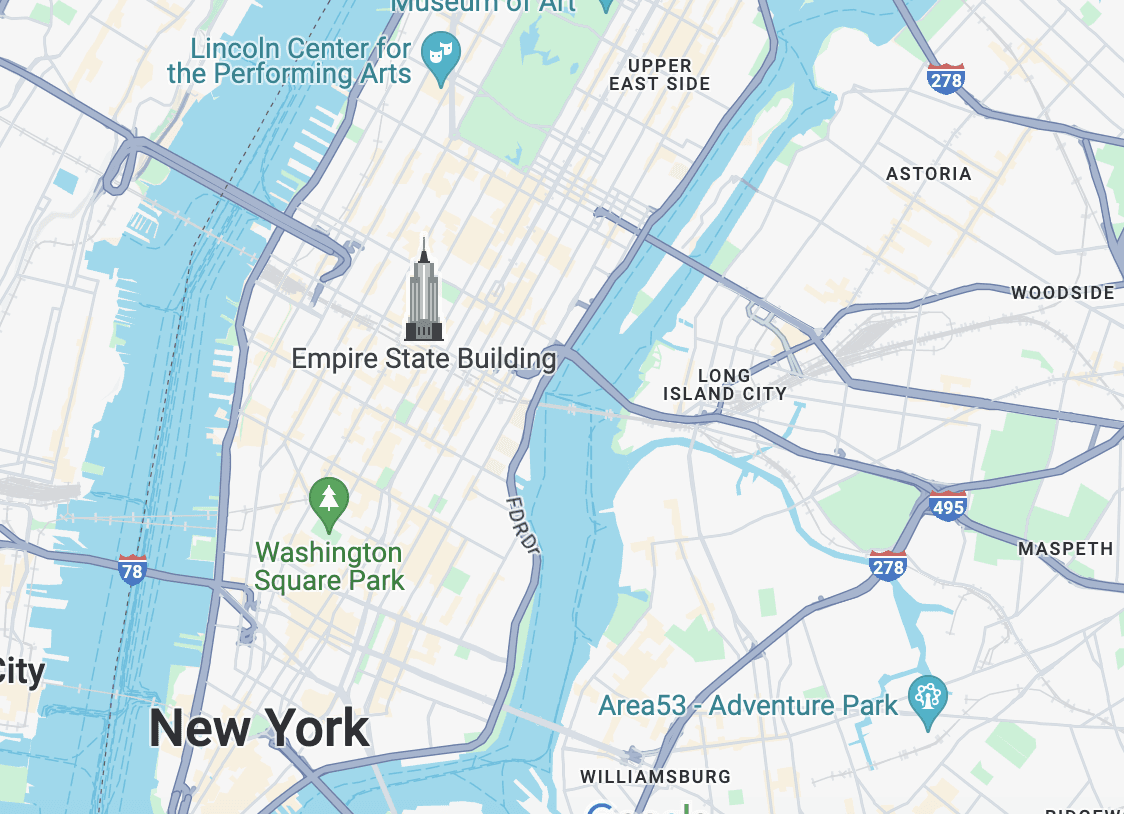
Rendering: Canvas
Google Maps/Google Maps API is indisputably the titan in the maps space. Adjacent to a massively popular consumer-facing product, the Google Maps API provides access to layered map data alongside personalizable features, such as creating custom markers and overlays. It’s built on HTML Canvas, affording it a snappy, responsive experience.
One of the core advantages of the Google Maps API ecosystem is the enormous community that embraces Google technologies. For instance, the community is responsible for helpful tools like this React auto-suggest library for prompting users with default suggestions.
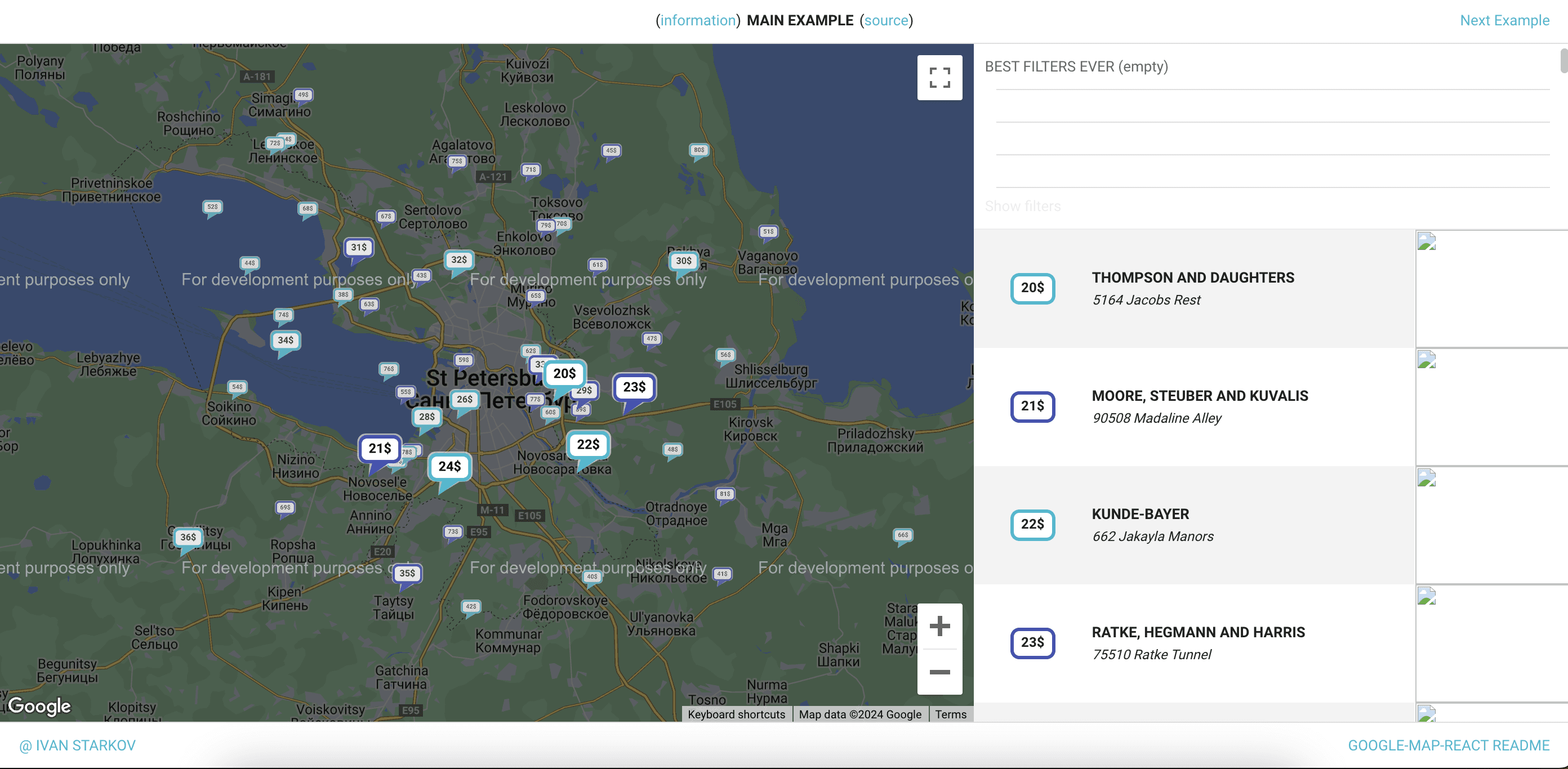
It’s worth establishing that every feature on Google Maps’ consumer product is also available to developers via a secondary API. These include features that take advantage of Google’s massive trove of data, such as Aerial Views and Photorealistic 3D Tiles, and plug-in–like secondary APIs such as Routes API, Places API, and the brand-new Environment API.
Of course, the Google Maps API isn’t the same thing as google-map-react. google-map-react is an open-source, unofficial React library that provides easy access to React components wrapped around the Google Maps API. One important clarification, though, is that while google-maps-react is free, community-driven, and open-source, its underlying product is proprietary, corporate-backed, and freemium: after 28,500 maploads, developers need to pay for the Google Maps API, with costs calculated via variable rates pegged to each OS, map tile type, and accessory feature.
Another important clarification is that google-map-react lacks one-to-one parity with the entire Google Maps API. As a community-driven library, it often trails features that are being actively, officially added to the Google Maps API platform. For developers using abstracted React packages who often care primarily about core features, this doesn’t tend to create too much trouble. But if you’re building applications that take advantage of niche features, using the Google Maps API raw dynamic import could be a better move.
The library has decent documentation (admittedly, just a fancy README) but fantastic adoption (nearly a quarter million weekly downloads!).
You can install google-map-react using a package manager like NPM or Yarn:
1npm install --save google-map-react
2#or
3yarn add google-map-react
Initializing a map is easy, where the GoogleMapReact
component needs to be invoked, with configurations passed as props:
1const handleApiLoaded = (map, maps) => {
2 // use map and maps objects
3};
4
5<GoogleMapReact
6 bootstrapURLKeys={{ key: /* YOUR KEY HERE */ }}
7 defaultCenter={this.props.center}
8 defaultZoom={this.props.zoom}
9 yesIWantToUseGoogleMapApiInternals
10 onGoogleApiLoaded={({ map, maps }) => handleApiLoaded(map, maps)}
11>
12 <AnyReactComponent
13 lat={59.955413}
14 lng={30.337844}
15 text="My Marker"
16 />
17</GoogleMapReact>
18
19// example courtesy of <https://www.npmjs.com/package/google-map-react>
Fundamentally, this is more of a question of when to use the Google Maps API. The Google Maps API can be a good fit for the following developers who:
- Are already immersed in Google’s ecosystem of developer tools
- Need to leverage up-to-date geolocation data
- Need to leverage routing algorithms
- Want to showcase a familiar experience to most users
Generally speaking, google-map-react and its underlying product are unopinionated and easy to learn. But they can be expensive, with Google Maps API notably raising its pricing 14x in recent years.
The community-driven, raw-HTML favorite
GitHub Stars: 4.9k
NPM weekly downloads: 267k
License: Hippocratic License
Rendering: HTML
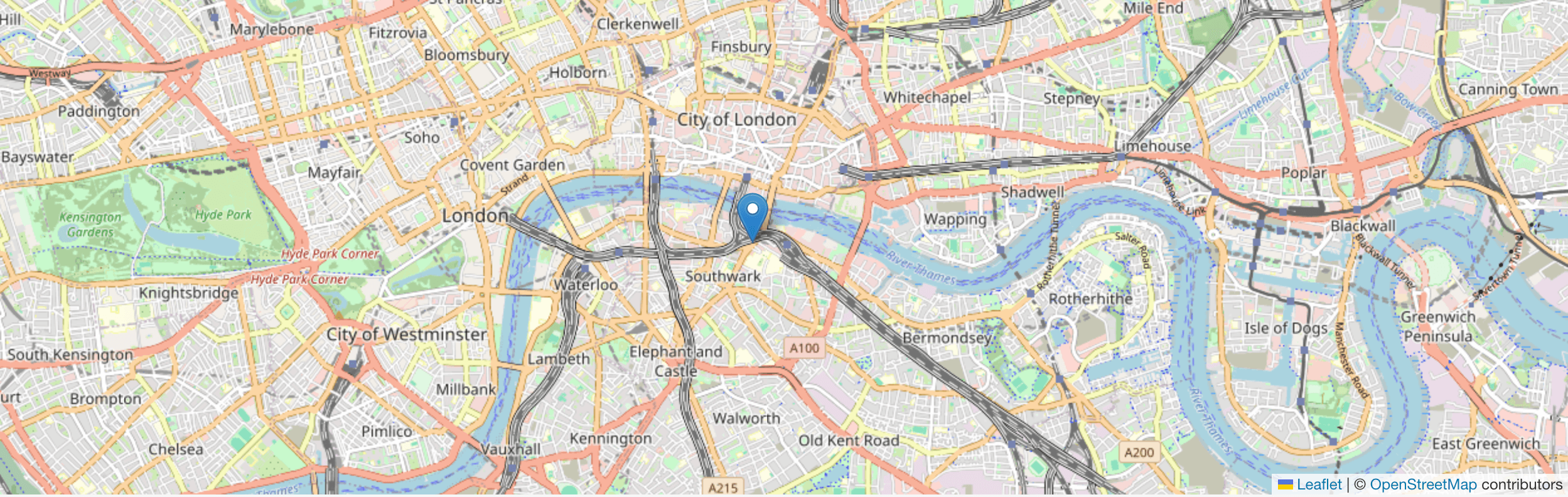
Leaflet is the leading unopinionated JavaScript library for maps. In this context, unopinionated means Leaflet is not bound to a specific map data set like Google Maps and Mapbox options are. Instead, Leaflet is a library that ingests any repository of map data (including proprietary data such as the aforementioned) and renders an interactive map.
Technically speaking, Leaflet is impressively simple. It boasts a tiny weight (42kB) without skimping on core mapping features. It also sports an *official* React plugin. It has a mature documentation hub with live preview editors, works across browsers, and is mobile-friendly. And, most notably, it works via … HTML!
That’s right. rReact-leaflet expects React to mount the MapContainer
but afterwards, handles rendering a map using raw HTML components. (That is, <a href>
, <div>
, and <img>
tags.) Of course, pure HTML rendering isn’t inherently a performance gain; here, it’s frankly a negative because of the slowness of browsers rendering new images on panning/zooming.
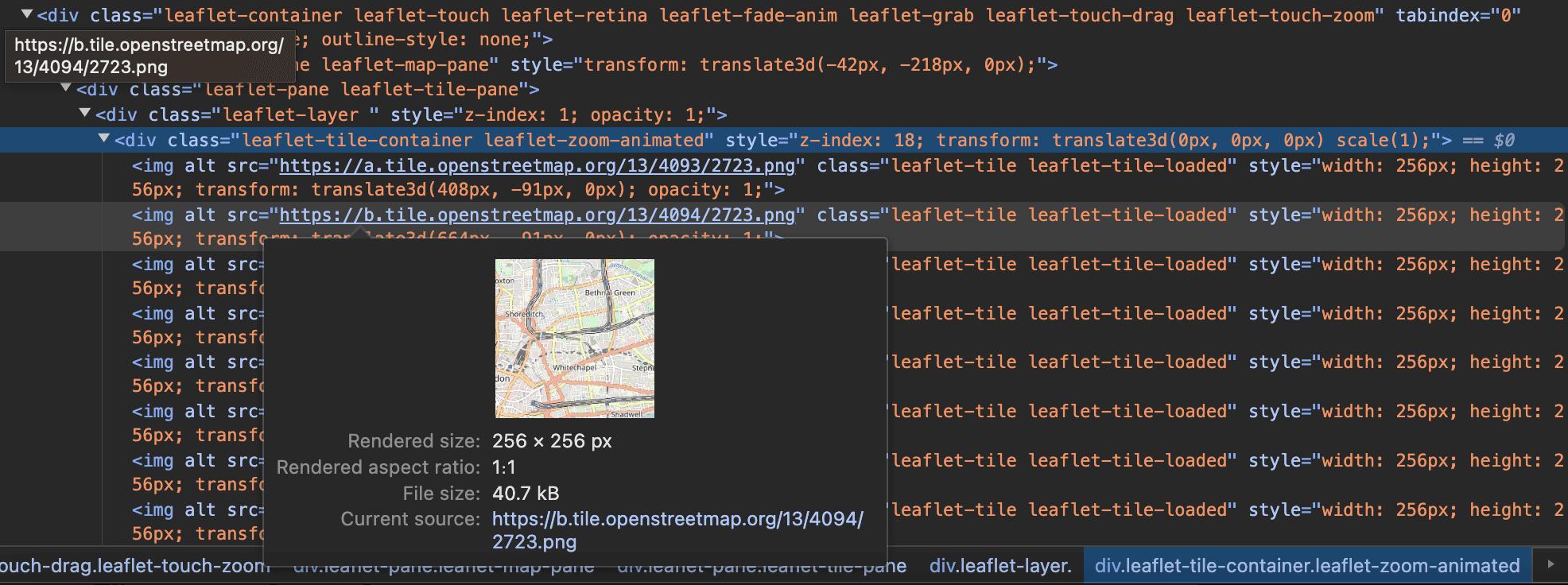
Leaflet supports zoom, custom markers, layers, and overlays. It easily integrates with open-source mapping data such as OpenStreetMap, and works with proprietary maps like Mapbox or even Google Maps—the latter made easy by this neat package.
One curious aspect of Leaflet is its license. While most libraries are either proprietary or open source under an MIT License or Apache License, react-leaflet is neither. Instead, react-leaflet uses the rather new Hippocratic License, which is not an open-source license according to the OSI. Why? Because the license stipulates that organizations that cause net social harm (citing examples such as militaries) are forbidden from using the technology. Unless your use case falls into those excluded categories, for a majority of readers, the react-leaflet library is practically open source (free to use, modify, and distribute).
You can install react-leaflet with a few approaches. You can use a package manager like NPM or Yarn:
1npm install react react-dom leaflet
2#or
3yarn add react react-dom leaflet
If you are using TypeScript, you can install the types via:
1npm install -D @types/leaflet
2#or
3yarn add -D @types/leaflet
Alternatively, you can use the package’s CDN directly:
1import {
2 MapContainer,
3 TileLayer,
4 useMap,
5} from '<https://cdn.esm.sh/react-leaflet>'
Leaflet is ideal for development teams that may prefer a configurable, community-driven product, specifically if developers:
- Want to use a custom map data set
- Are price-conscious
- Want to utilize only raw HTML rendering
- Want an officially supported React package
Generally speaking, react-leaflet and Google Maps API are often regarded as the top two leaders in the map library space. That being said, don’t discount our next choice: react-map-gl/Mapbox.
The designer’s favorite, powered by WebGL
GitHub Stars: 7.5k
NPM weekly downloads: 302k
License: MIT
Rendering: WebGL
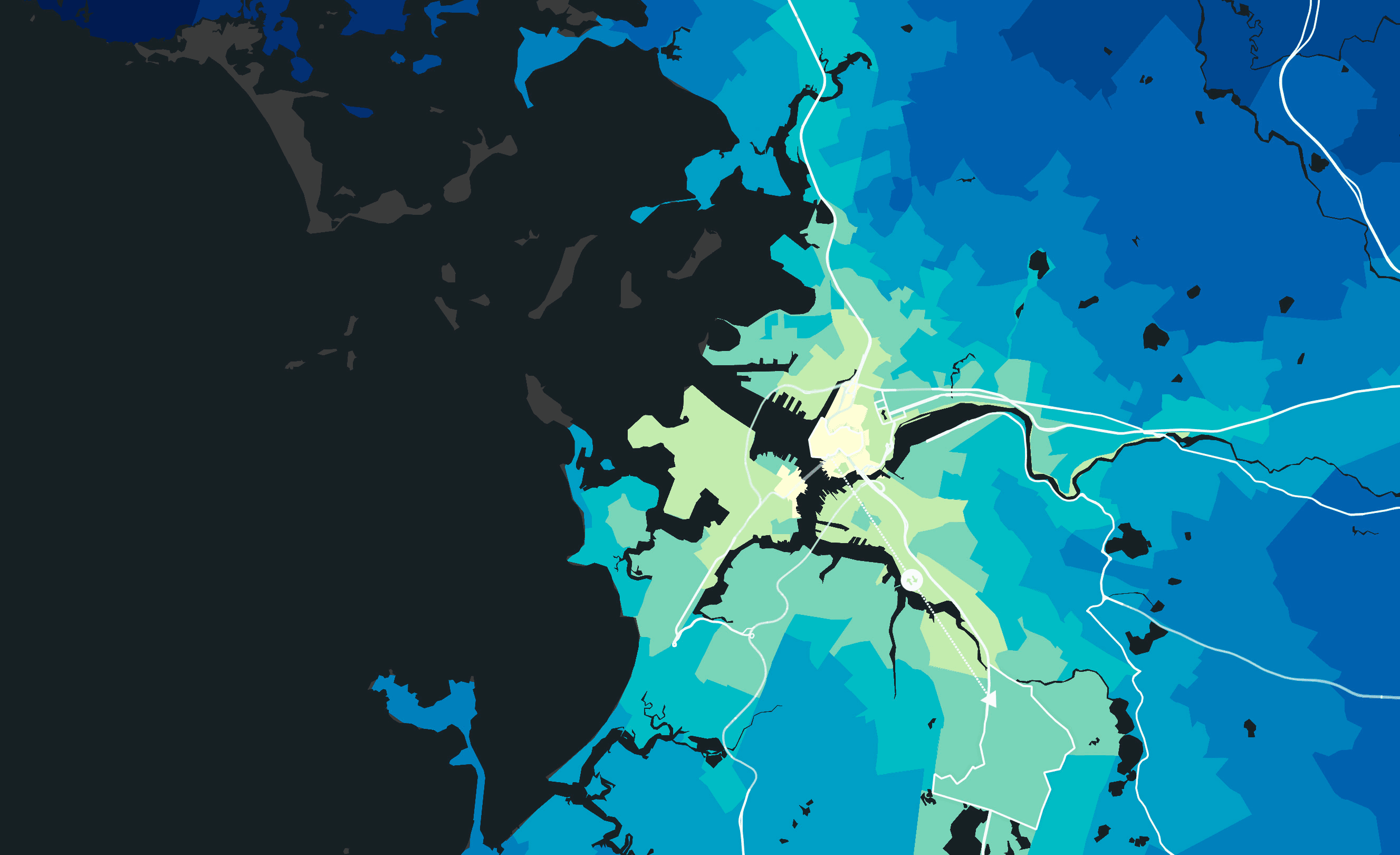
react-map-gl is a React wrapper around Mapbox GL. Mapbox GL, meanwhile, is a (vanilla) JavaScript SDK for Mapbox. And, since we’re going down the list, what about Mapbox itself? That’s the biggest competitor to the Google Maps API.
First off, Mapbox’s maps are beautiful. The company is dedicated to creating polished, interactive maps that often look like art pieces. Google might be the titan when it comes to map data, but Mapbox’s 3D maps are crisp, its terrain maps are textured, and its map projections are snappy.
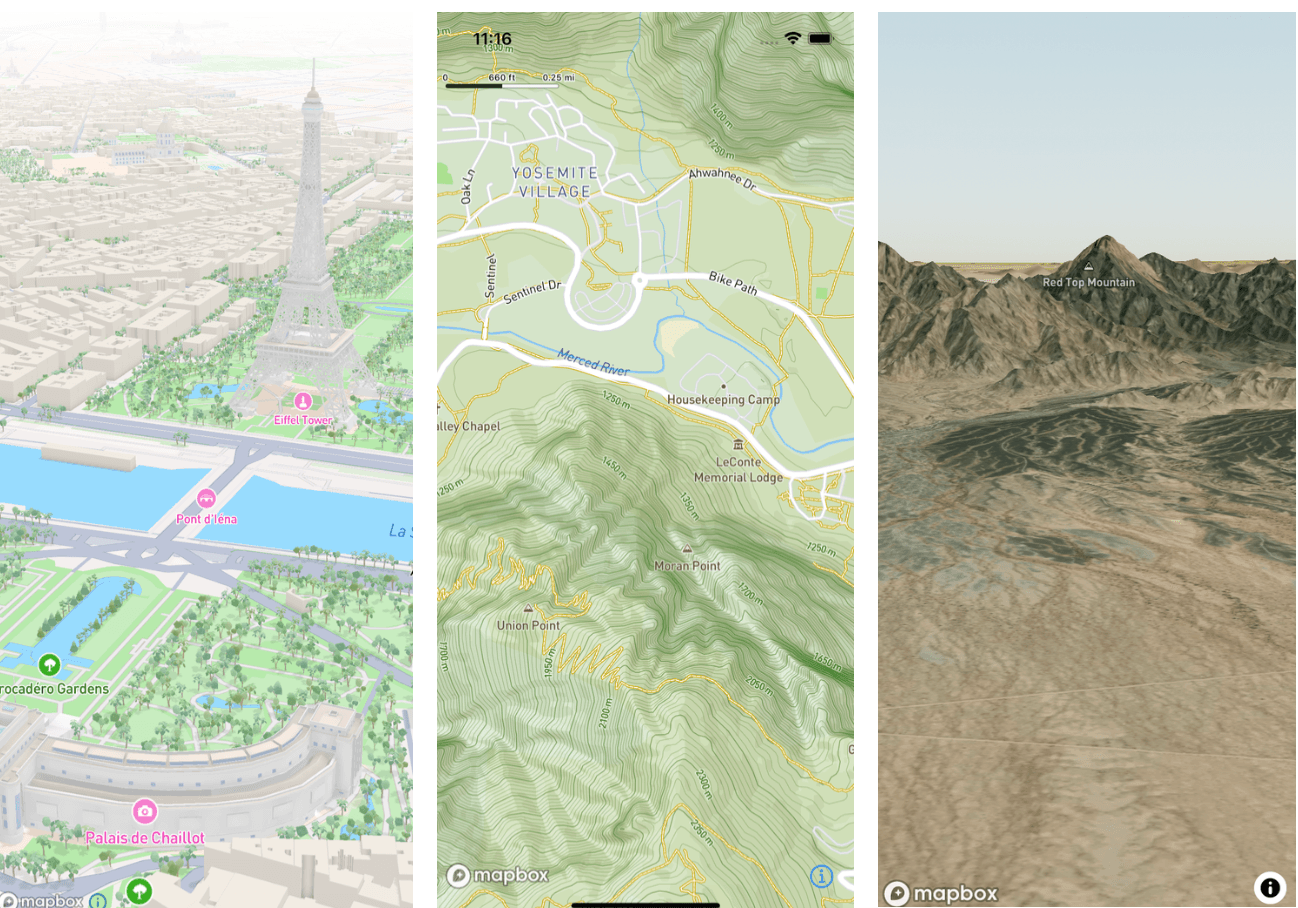
Mapbox’s aesthetic prowess is made possible by its utilization of WebGL, a JavaScript rendering environment for 3D graphics that can produce high-resolution, performant visuals. This is particularly obvious when moving around Mapbox maps—the browser has no issue displaying these video-game-like graphics.
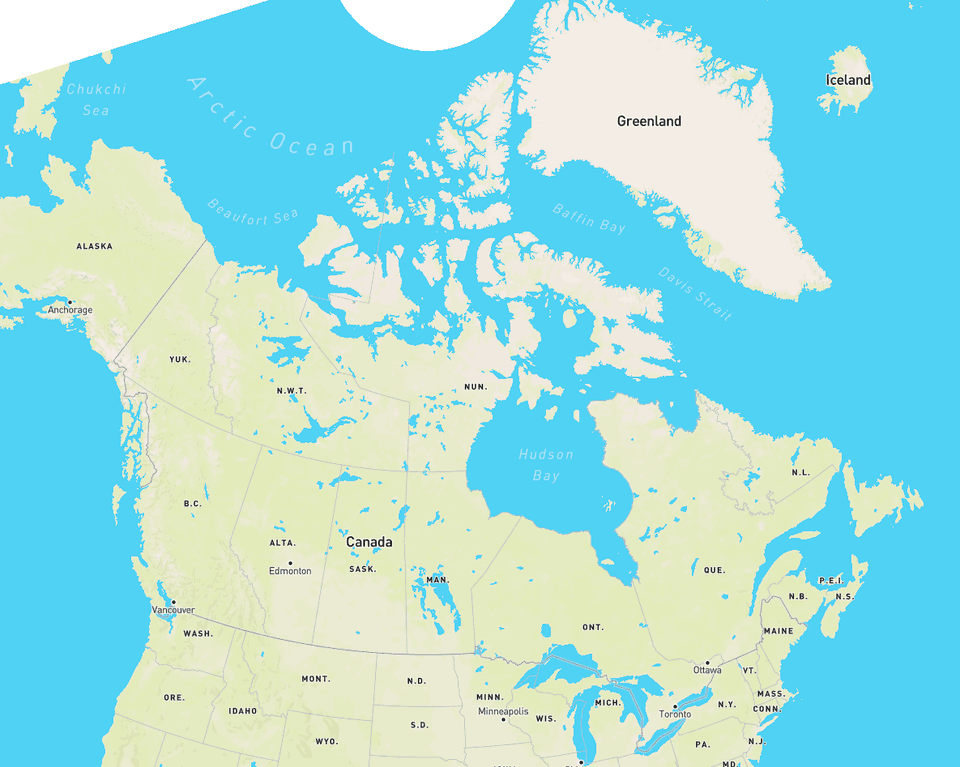
Mapbox also supports complex features such as navigation (corollary to Google Maps API’s Routes API) and powerful Tileset features that enable seamless zoom.
You can install react-map-gl and its dependencies like mapbox-gl via NPM:
1npm install --save react-map-gl mapbox-gl @types/mapbox-gl
Then, you can initialize react-map-gl by using the Map
component:
1import * as React from 'react';
2import Map from 'react-map-gl';
3
4function App() {
5 return (
6 <Map
7 mapboxAccessToken="<Mapbox access token>"
8 initialViewState={{
9 longitude: -122.4,
10 latitude: 37.8,
11 zoom: 14
12 }}
13 style={{width: 600, height: 400}}
14 mapStyle="mapbox://styles/mapbox/streets-v9"
15 />
16 );
17}
18
19#example courtesy of <https://visgl.github.io/react-map-gl/docs/get-started>
react-map-gl’s visualization-heavy focus is best explained by its story. The library was initially developed by Uber’s team—not for the Uber app, but for various internal geographic analytics tools. Mapbox was designed to depict a metadata-heavy map. This type of library will work best for builders and teams who:
- Want to leverage 3D graphs
- Care about the aesthetic of their end maps
- Want a brandable map experience
- Need to display the entire globe, the atmosphere, or any custom projections
Mapbox is an enterprise-friendly product but has pricing that’s friendly to smaller developers: The first 50,000 maploads on browsers are included in the free tier, beating Google’s 28,500 threshold. Similarly to Google, Mapbox’s rates are specific to each feature.
The SVG-driven simplifier’s favorite
GitHub Stars: 3.0k
NPM weekly downloads: 69.8k
License: MIT
Rendering: SVG
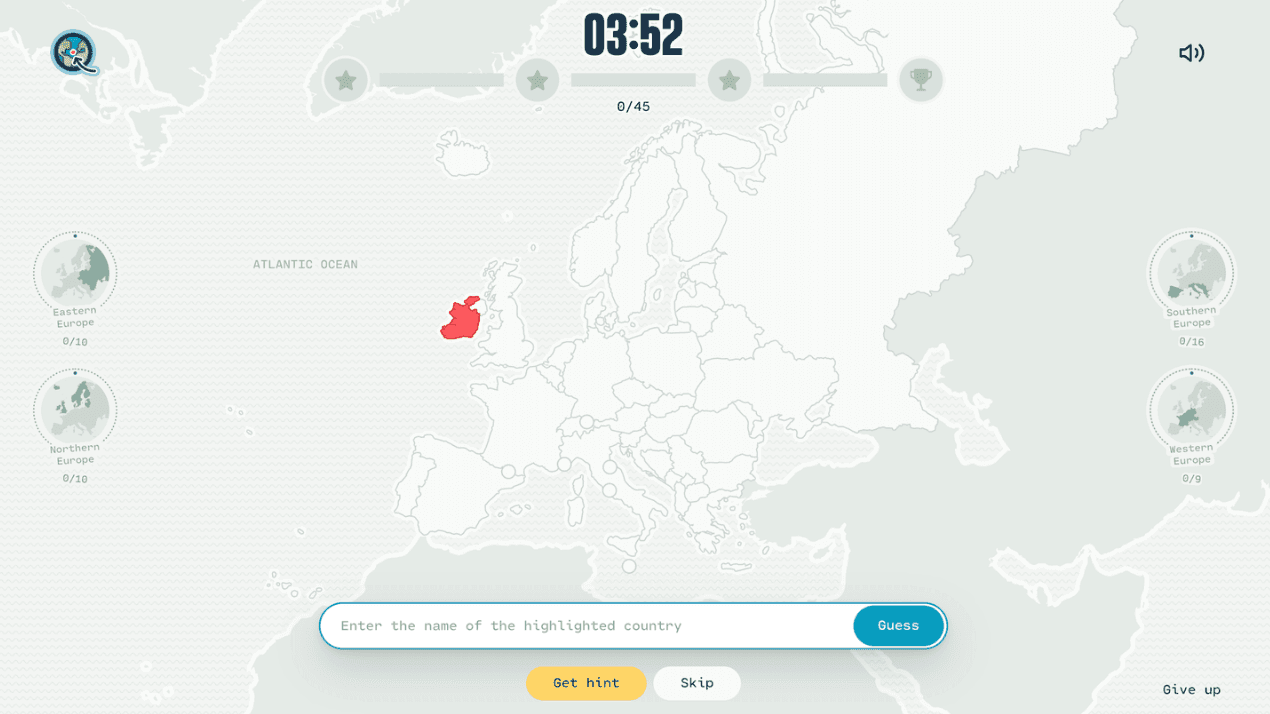
react-simplemaps was built to use a similar design language as other major React libraries, including react-annotation, react-spring, react-tooltip, styled-components, and victory. Practically, react-simple-maps is a thin wrapper around d3-geo and topojson.
Because of the limitations (but also simplicity) of SVGs, react-simple-maps is great for maps that aren’t meant to be super interactive and are instead displaying information. It’s fantastic, for instance, for highlighting countries or regions.
You can install react-simple-maps via NPM or Yarn:
1npm install --save react-simple-maps
2#
3yarn add --save react-simple-maps
Example courtesy of react-simple docs.
1import React from "react"
2import { ComposableMap, Geographies, Geography } from "react-simple-maps"
3
4const geoUrl =
5 "<https://raw.githubusercontent.com/deldersveld/topojson/master/world-countries.json>"
6
7export default function MapChart() {
8 return (
9 <ComposableMap>
10 <Geographies geography={geoUrl}>
11 {({ geographies }) =>
12 geographies.map((geo) => (
13 <Geography key={geo.rsmKey} geography={geo} />
14 ))
15 }
16 </Geographies>
17 </ComposableMap>
18 )
19}
react-simple-maps is built for developers who want a simple map experience. If a developer needs a map that’s going to be easy on the browser, is primarily a visual aid, and expects topojson data, then react-simple-maps is ideal.
In summary, react-simple-maps is for builders and teams who:
- Want a browser-friendly rendering experience
- Need to use maps as a visual aid rather than for a heavily interactive experience
- Want something that feels similar to other common react libraries
The … hipster favorite?
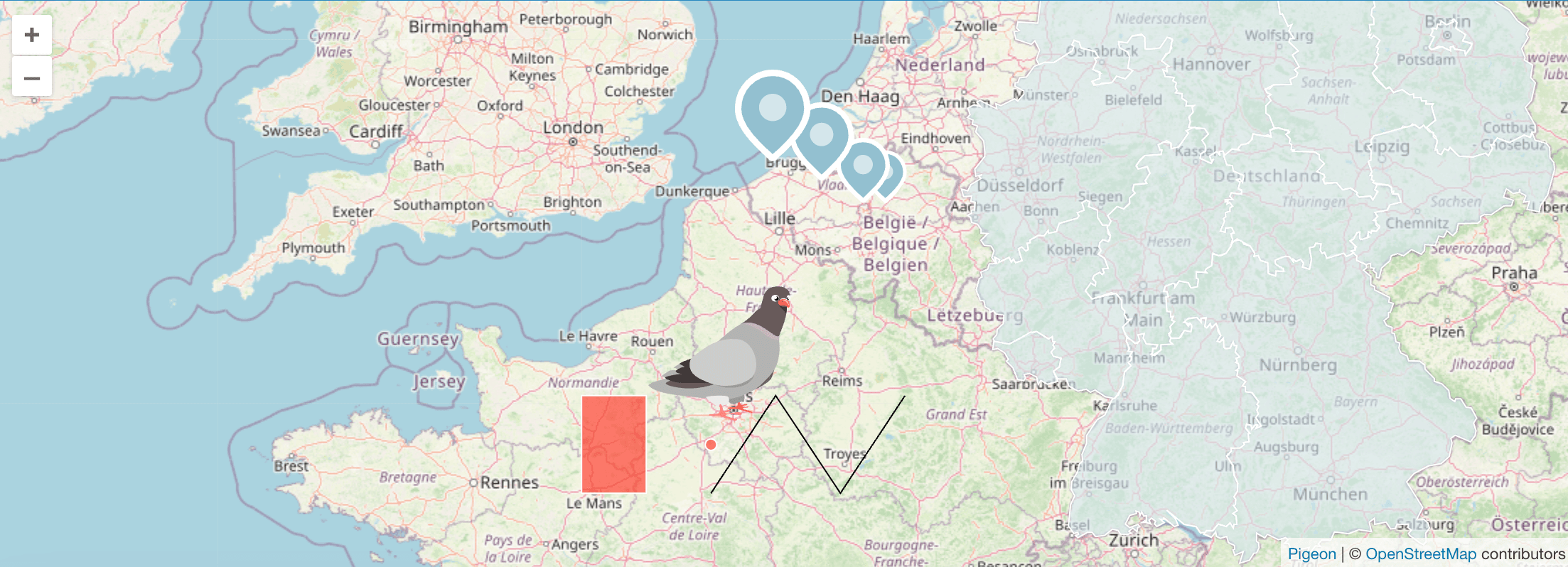
GitHub Stars: 3.4k
NPM weekly downloads: 19k
License: MIT
Rendering: HTML
pigeon-maps was on a previous version of this list but was moved to honorable mention due to lower NPM weekly downloads. Regardless, it deserves a mention because of its core differentiator: it doesn’t rely on external dependencies. (Also, a pigeon.)
Pigeon touts this accolade as a package size benefit, with its documentation citing Google Maps and react-leaflet unpacking at 200kB and 140kB, respectively. Today, however, Google Maps has compressed to 111kB and react-leaflet to 50kB. Meanwhile, Pigeon sits at … 229kB. 🤷
Perhaps time hasn’t been on its side, but pigeon-maps remains an interesting project. Pigeon imports maps from MapTiler, OpenStreetMap, and Stamen (all open-source projects). Like the other libraries, it supports markers, interactive movements, overlays, and event handling. Predictably, it doesn’t support some of the more complex features available in react-leaflet or Google Maps API, such as panes or tooltips.
Pigeon started as a performance-driven in-house project for Apprentus when Google’s loading time wasn’t cutting it. These days, that performance delta is less glaring. To the credit of Pigeon, though, its weekly download rate, albeit humble, has remained consistent.
There’s a lot for builders and teams to consider when developing maps. For developers that want a familiar interface and already like the Google Maps interface, google-map-react can be a great fit. For developers who want a community-driven, unopinionated library, react-leaflet is often a good choice. And for developers that want to take advantage of a performant set of specialized map interfaces, Mapbox’s react-map-gl might be the strongest candidate.
Overall, there’s no one-size-fits-all solution because not all mapping tasks have the same priorities. Some teams, though, may prefer a pre-built, managed map component to make spin up extra streamlined. If that sounds like you, sign up for a free Retool account to try the managed Mapbox component today.
Reader