It's December for developers - a time of rest, reflection, and (sometimes!) quiet days at work to write a lot of code. You might even find yourself with extra time to sharpen up your developer skills, or learn brand new ones. A great way to do both is to participate in Advent of Code, featuring daily code challenges that will put your programming skills to the test.
In this post, we will orient you a bit on how Advent of Code works, show how you can participate in Advent of Code, and finally (SPOILERS) share the solution to the Day 1 challenge for Advent of Code 2022 in JavaScript.
Every day leading up to December 25th, a new code challenge is unlocked on the Advent of Code website. Creator Eric Wastl weaves a charming story that surfaces a holiday code challenge, which developers can tackle using the programming language of their choice.
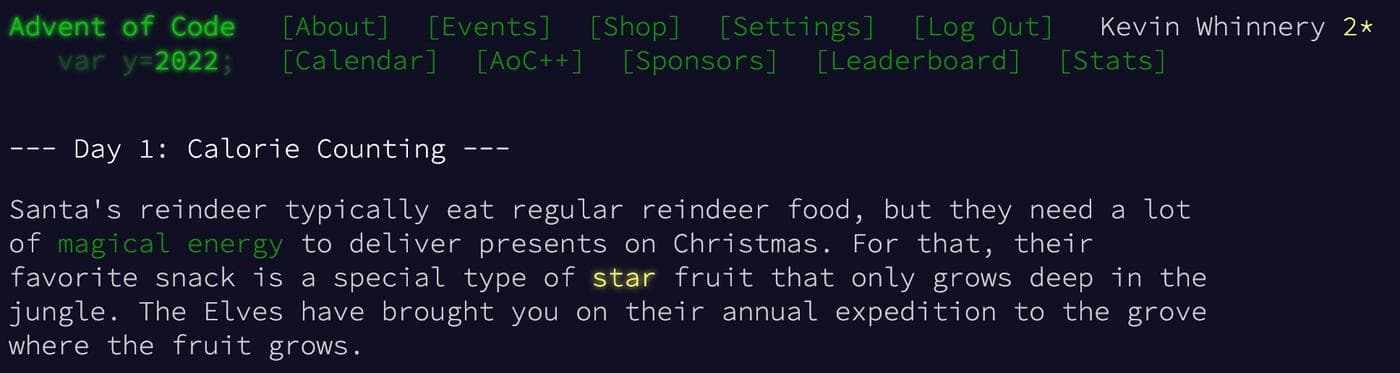
The programming challenge requires developers to use input text that is dynamically generated for them, and write logic that processes that input according to a set of criteria described in the story. Every day, there are two challenges of increasing difficulty that use the same data set.
You can use any programming environment you like to complete Advent of Code challenges. You can download your unique input text, and process it using the tools of your choice. If you're a JavaScript developer (or would like to become one!) you can also complete Advent of Code using the JavaScript development environment in Retool. I've built a small test harness that you can use to paste in your dynamic puzzle input, and write JavaScript code to parse it and output your answers in a UI.
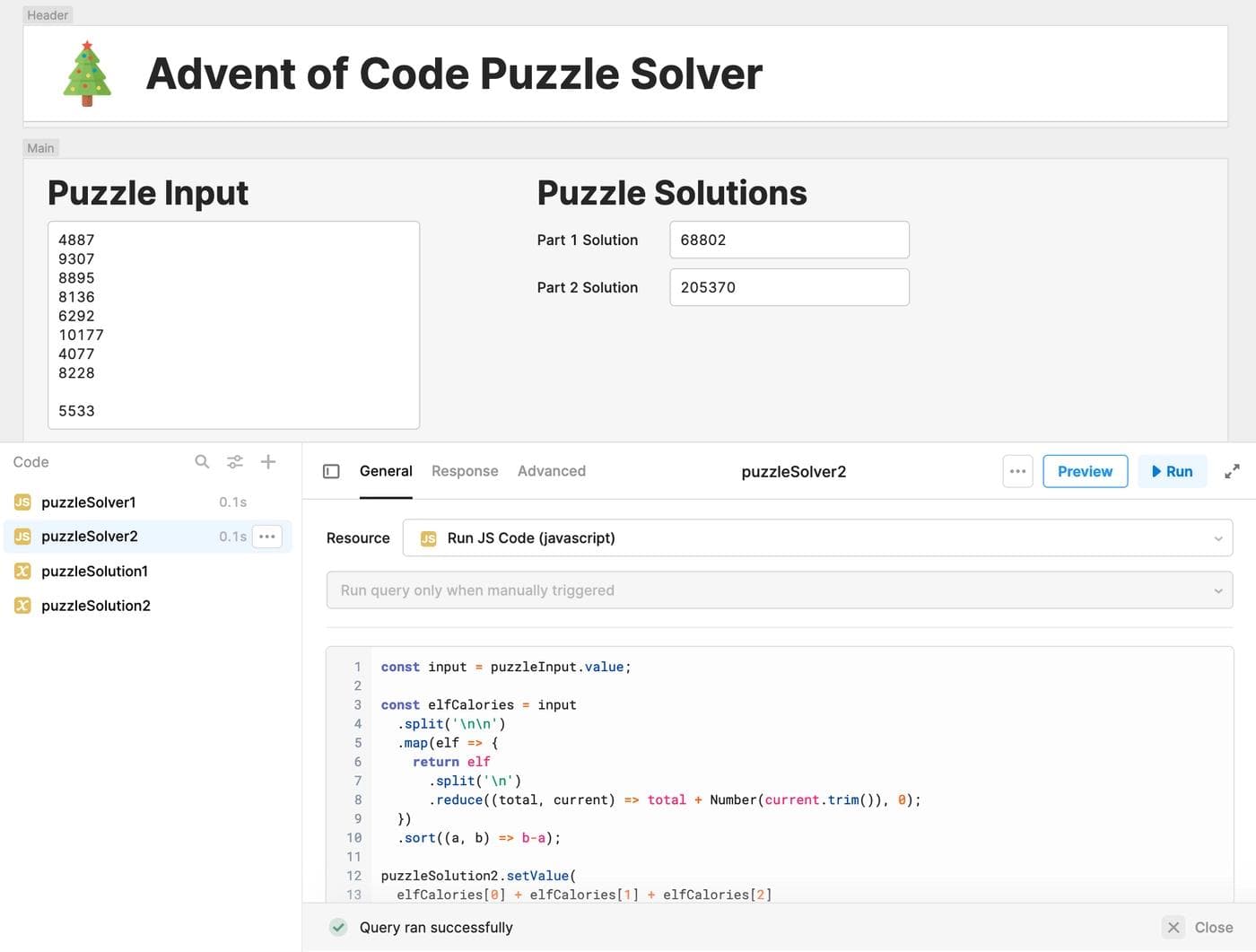
Here's how you can get this dev environment going in just a few minutes. If you already have a JavaScript environment that you like to use, feel free to scroll down to the code solutions below these setup steps.
1.) Sign up for a free Retool account
2.) Create a new Retool application using this JSON file as a template.
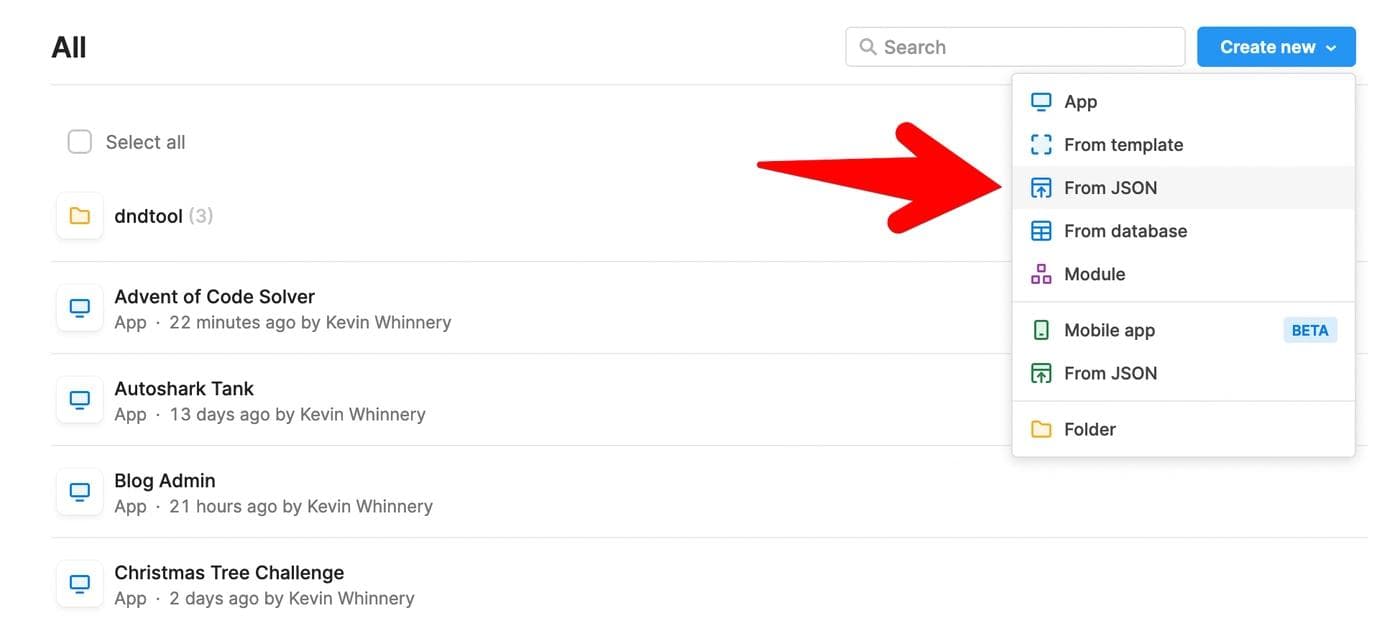
3.) After importing the app, you should be viewing it in "edit mode". Inside the app, there is a text field underneath the heading Puzzle Input. This is where you can paste your unique puzzle input from Advent of Code.
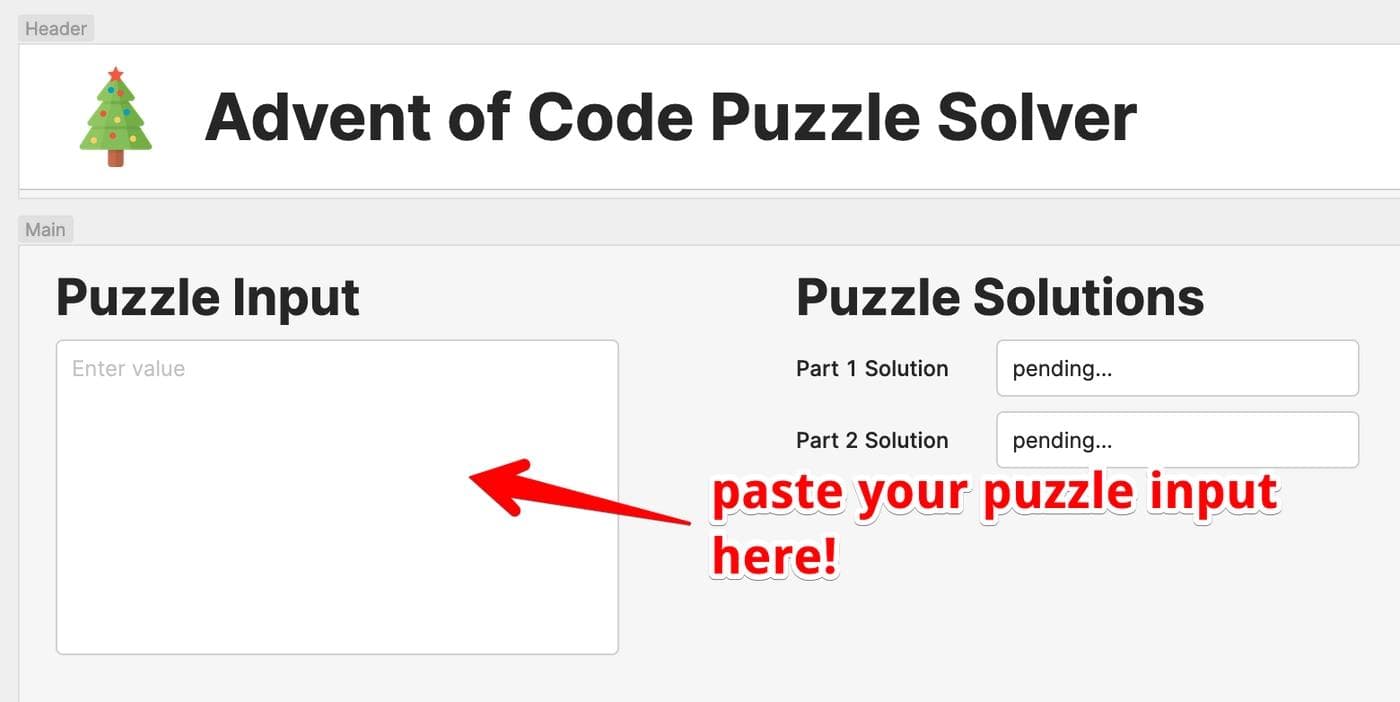
4.) Write JavaScript code to solve the puzzle using the two preconfigured JavaScript queries (these are Retool's way of letting you write JavaScript code to handle events in a normal Retool app).
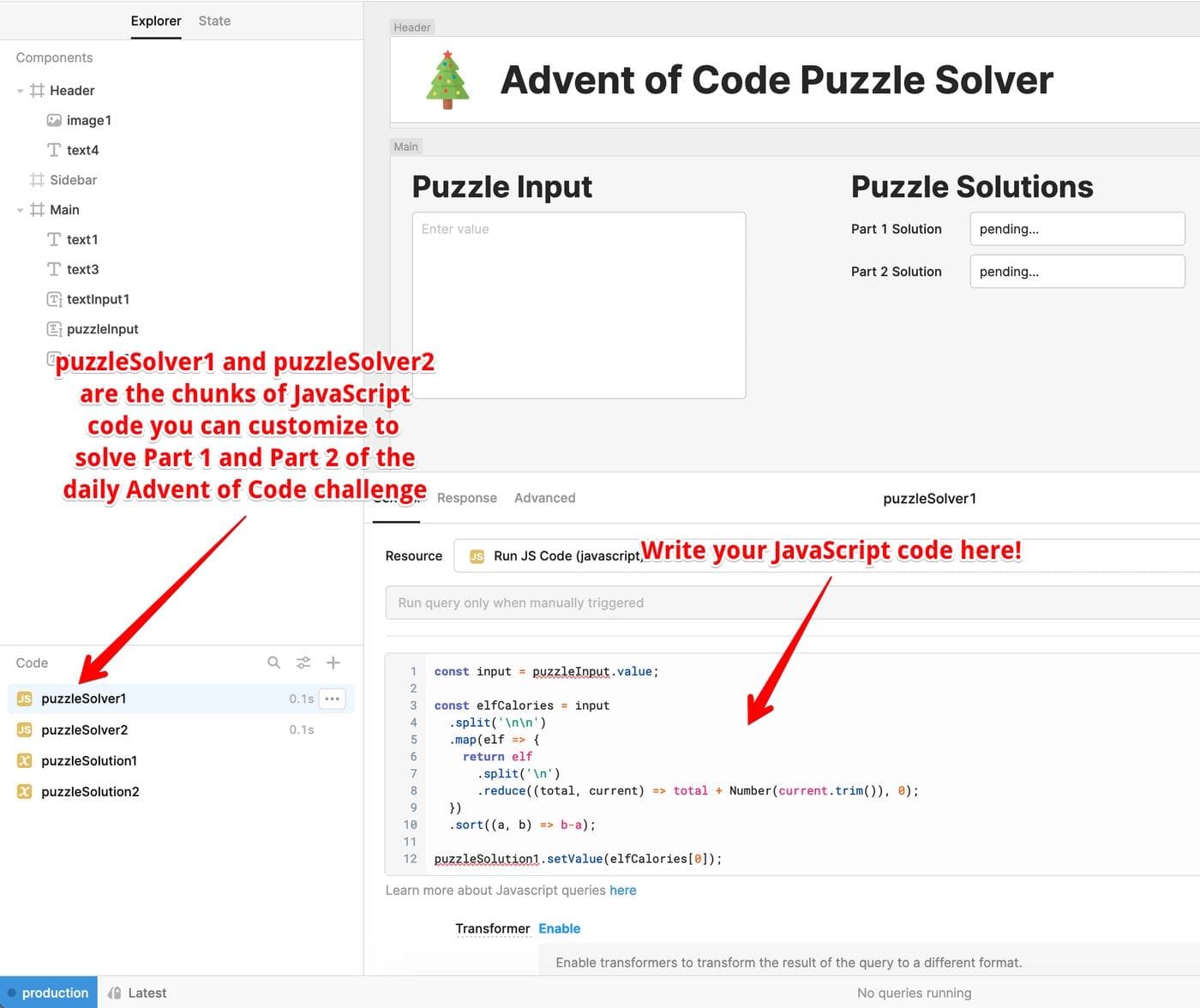
5.) Run the JavaScript code for either puzzleSolver1 or puzzleSolver2 once you have pasted in your puzzle input text. The code samples are set up to render the results required for the puzzle in the input text fields labeled Part 1 Solution or Part 2 Solution, respectively.
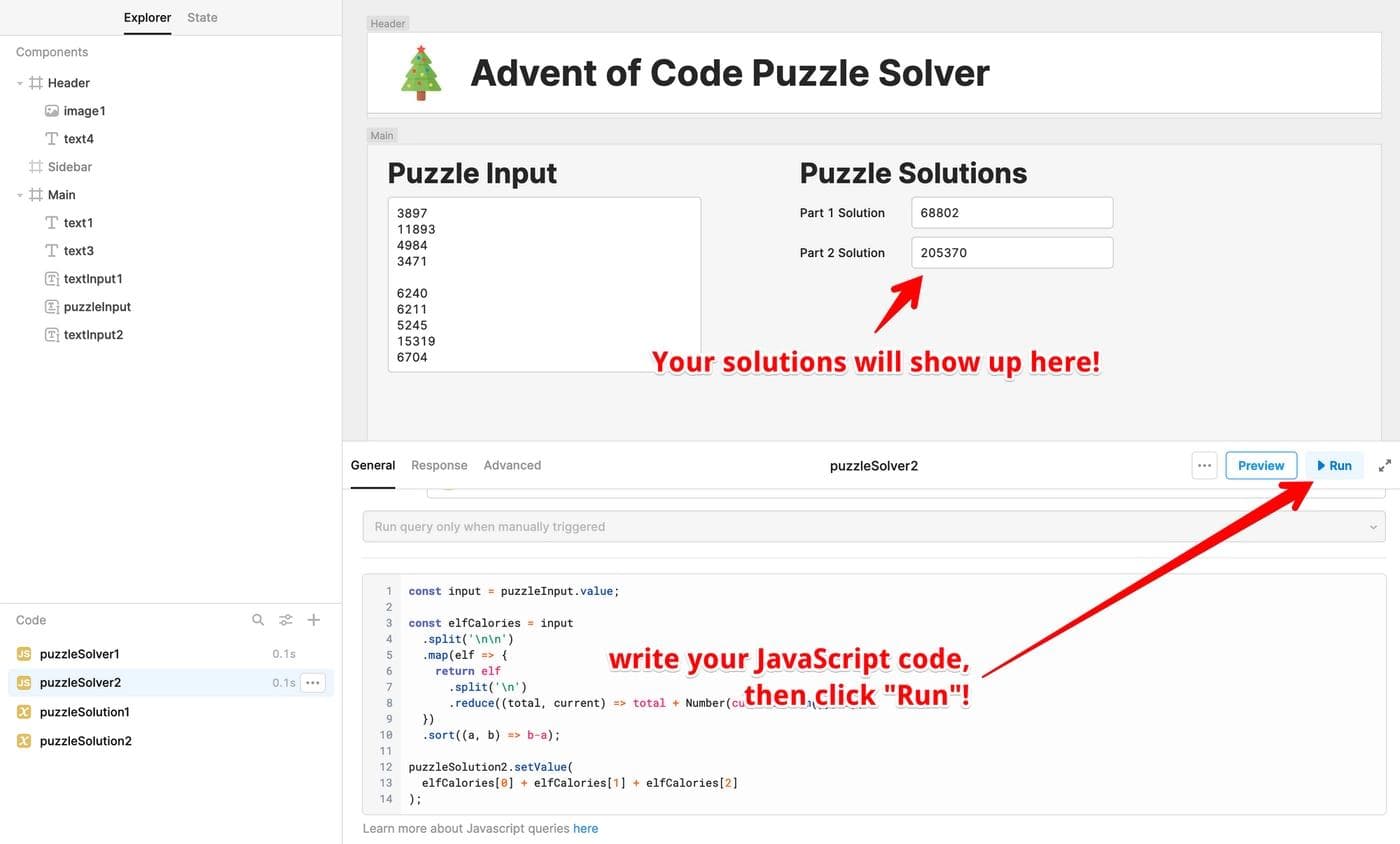
After computing your solutions, you can copy and paste these values into the Advent of Code UI to check your work. Now that we have an IDE set up to process our input from Advent of Code, let's check out the solutions for Day 1 2022!
Today's challenge requires you to process a block of text that is delimited by newline characters - "\n". These invisible characters instruct a computer to insert a new line when rendering text for human eyes. These characters also serve as delimiters that we can use as developers to split up the input text and work with each chunk of data.
This challenge gives us groups of numbers representing the calories of food items carried by elves on a magical quest. Blank lines indicate where the list of food items carried by one elf ends, and where the next begins. Here's an example.
1 2 3 4 5 6 7
1000 4000 1200 500 3000
This example represents three elves - the first has food items representing 5,000 calories, the second 1,700 calories, and the third 3,000 calories.
Part One of the challenge asks us to total up all the calories each individual elf is carrying, and provide the highest total amount of calories carried by a single elf. Using the example data above, the answer would be 5,000, the largest total calorie amount any elf is carrying.
To calculate this value, we'll need to do some JavaScript string manipulation and array processing. Here is the full solution (embedded in the Retool example app as the puzzleSolver1 JavaScript query) for the first challenge. Read the comments below to understand what's happening at each step.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28
// 1.) First, we get the string input for the puzzle const input = puzzleInput.value; // 2.) This chain of code chunks up the input data and ultimately // produces an ordered array of the total calories carried by // each elf in the party const elfCalories = input // 2a.) create an array with each elf's calories - a double // newline (a blank line) indicates a break between the // calorie values listed for each elf .split('\n\n') // 2b.) aggregate the calories for each elf into an array // of single numbers using Array.map .map(elf => { // 2c.) Combine all the calorie values for an individual elf // to a single number using Array.reduce return elf .split('\n') .reduce((total, current) => total + Number(current.trim()), 0); }) // 2d.) Sort the resulting array from highest to lowest calories .sort((a, b) => b-a); // 3.) Display the value of the highest calorie elf in the text field puzzleSolution1.setValue(elfCalories[0]);
Part Two of the challenge is very similar, but instead of asking for the single highest calorie value, it asks for the sum of the top three highest calorie-carrying elves. So instead of showing the highest number, we add the top three. Here is the code you'll find in the puzzleSolver2 JavaScript query.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
const input = puzzleInput.value; const elfCalories = input .split('\n\n') .map(elf => { return elf .split('\n') .reduce((total, current) => total + Number(current.trim()), 0); }) .sort((a, b) => b-a); puzzleSolution2.setValue( // The solution is the sum of the first three values in the // sorted array! elfCalories[0] + elfCalories[1] + elfCalories[2] );
And there you have it! You are well on your way to earning all the stars necessary to ace Advent of Code in 2022.
What did you think of this solution? Is there a more elegant way to process the data? When writing algorithms of this type, there's almost always a better, faster way of doing things. If you have any questions, comments, or improvements, please let me know. Have fun with Advent of Code!
Reader