One important skill for React users is to be able to create a navigation bar (navbar). You have multiple options for doing so, especially if you pair React with another free and open source CSS framework, such as Bootstrap or Tailwind CSS.
Bootstrap was developed as a framework to provide DRYer code and consistency across internal developer tools. Before this, projects relied on multiple libraries, which could lead to cluttered codebases. Bootstrap contains CSS and, optionally, JavaScript-based design templates for typography, forms, buttons, navigation, and other UI components. Numerous web applications rely on Bootstrap, including Apple Maps Connect.
Tailwind CSS scans HTML files, JavaScript components (and other templates for class names), generates the corresponding styles, and writes them to a static CSS file for easy readability.
In this tutorial, you're going to create a list navbar in React using Bootstrap and Tailwind.
Here’s what you’ll need for this project:
- Some knowledge of CSS
- Some knowledge of React
- npm installed on your local machine
- Head to Bootstrap’s Getting Started page.
1
npm install bootstrap
- Scroll down to Package managers for npm installation since this is a React project.
1
npm install react-bootstrap bootstrap@5.1.3
- Go to the Tailwind CSS documentation.
- Install Tailwind CSS using npm or yarn (check the docs for instructions):
1
npm install -D tailwindcss
- Create a file called
tailwind.config.js
once the package is installed:
1
npx tailwindcss init
- Configure your template paths. You can check examples in the documentation.
- Add the Tailwind directives to your main CSS file, usually the
index.css
file. These include the following:
1 2 3
@tailwind base; @tailwind components; @tailwind utilities;
- Start the Tailwind CLI build process:
1
npx tailwindcss -i ./src/<your main css file>.css -o ./dist/output.css
- Wait to receive the
Done
message in your terminal.
If you prefer, you can follow along with this GitHub repo.
Otherwise, create a React app if you haven’t already. In your terminal, run this:
1
npx create-react-app <your app name>
While you can create your navbar from scratch using the Bootstrap docs, Bootstrap provides a library of free UI components, including navbars with a navbar toggle option. Additionally, you can leverage the power of React hooks or custom hooks to enhance the functionality of the navbar.
- Head to the Getting started section.
- Go to the Navbar section and select a component. Use the Copy button on the top-right corner to copy it.
Below is the one used in the repository:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58
<nav className="navbar navbar-expand-lg navbar-light bg-light"> <a className="navbar-brand" href="#"> Navbar </a> <button className="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNavDropdown" aria-controls="navbarNavDropdown" aria-expanded="false" aria-label="Toggle navigation" > <span className="navbar-toggler-icon"></span> </button> <div className="collapse navbar-collapse" id="navbarNavDropdown"> <ul className="navbar-nav"> <li className="nav-item active"> <a className="nav-link" href="#"> Home <span className="sr-only">(current)</span> </a> </li> <li className="nav-item"> <a className="nav-link" href="#"> Features </a> </li> <li className="nav-item"> <a className="nav-link" href="#"> Pricing </a> </li> <li className="nav-item dropdown"> <a className="nav-link dropdown-toggle" href="#" id="navbarDropdownMenuLink" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false" > Dropdown link </a> <div className="dropdown-menu" aria-labelledby="navbarDropdownMenuLink"> <a className="dropdown-item" href="#"> Action </a> <a className="dropdown-item" href="#"> Another action </a> <a className="dropdown-item" href="#"> Something else here </a> </div> </li> </ul> </div> </nav>
- Go to your React application and create a
src/Navigation
folder. - Create a
src/Navigation/Navbar.js
file inside thesrc/Navigation
directory. - Create an empty navbar function in your file:
1 2 3 4 5 6
import React from "react"; const Navbar = () => { return () } export default Navbar
Paste in the Bootstrap CSS boilerplate navigation menu, as well as the navbar contents you already copied over:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70
import React from "react"; const Navbar = () => { return ( <nav className="navbar navbar-expand-lg navbar-light bg-light"> <a className="navbar-brand" href="#"> Navbar </a> <button className="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarNavDropdown" aria-controls="navbarNavDropdown" aria-expanded="false" aria-label="Toggle navigation" > <span className="navbar-toggler-icon"></span> </button> <div className="collapse navbar-collapse" id="navbarNavDropdown"> <ul className="navbar-nav"> <li className="nav-item active"> <a className="nav-link" href="#"> Home <span className="sr-only">(current)</span> </a> </li> <li className="nav-item"> <a className="nav-link" href="#"> Features </a> </li> <li className="nav-item"> <a className="nav-link" href="#"> Pricing </a> </li> <li className="nav-item dropdown"> <a className="nav-link dropdown-toggle" href="#" id="navbarDropdownMenuLink" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false" > Dropdown link </a> <div className="dropdown-menu" aria-labelledby="navbarDropdownMenuLink" > <a className="dropdown-item" href="#"> Action </a> <a className="dropdown-item" href="#"> Another action </a> <a className="dropdown-item" href="#"> Something else here </a> </div> </li> </ul> </div> </nav> ); }; export default Navbar;
- Head to your
App.js
and importsrc/Navigation/Navbar.js
.
Be sure to also import bootstrap/dist/css/bootstrap.min.css
since this code file contains the configuration for Bootstrap React CSS.
1 2 3 4 5 6 7 8 9 10
import "bootstrap/dist/css/bootstrap.min.css"; import Navbar from "./Navigation/Navbar.js"; function App() { return ( <div> <Navbar /> </div> ); }
You’ve now successfully built a navigation bar using Bootstrap.
- Run
npm start
to see it in action.
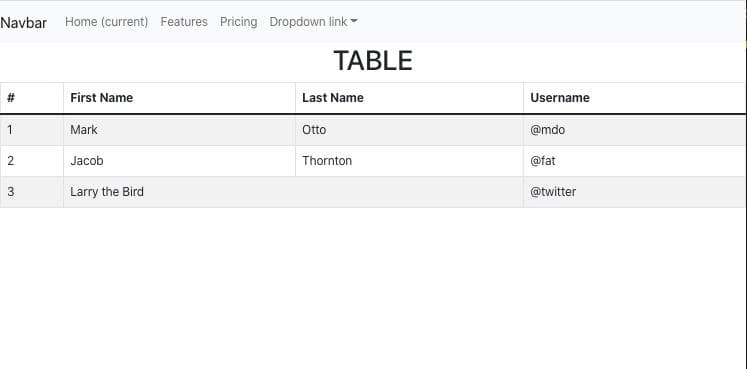
You can also head back to the Bootstrap docs to customize it further.
If you’d rather follow along, check the GitHub repo.
Otherwise, create a React app, if you haven’t already. Go to your terminal and use this command:
1
npx create-react-app <your app name>
While you can create a navbar using the Tailwind CSS docs, Tailwind provides a library of free UI components, including navbars. (There are plenty of other free Tailwind UI component libraries available, including daisyUI).
- Go to Tailwind UI, then to the Navbars section. Pick one navbar, click the HTML button on the top right, and select React from the drop-down menu.
- Find the toggle with an eye (Preview) and two code block arrows (Code) next to the drop-down menu. Switch the toggle to the arrows (Code), and you’ll see the boilerplate code for your Tailwind CSS navbar. Copy it.
Here’s the one used in the example repository:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168
import { Fragment } from "react"; import { Disclosure, Menu, Transition } from "@headlessui/react"; import { BellIcon, MenuIcon, XIcon } from "@heroicons/react/outline"; const navigation = [ { name: "Dashboard", href: "#", current: true }, { name: "Team", href: "#", current: false }, { name: "Projects", href: "#", current: false }, { name: "Calendar", href: "#", current: false }, ]; function classNames(...classes) { return classes.filter(Boolean).join(" "); } export default function Navbar() { return ( <Disclosure as="nav" className="bg-gray-800"> {({ open }) => ( <> <div className="max-w-7xl mx-auto px-2 sm:px-6 lg:px-8"> <div className="relative flex items-center justify-between h-16"> <div className="absolute inset-y-0 left-0 flex items-center sm:hidden"> {/* Mobile menu button*/} <Disclosure.Button className="inline-flex items-center justify-center p-2 rounded-md text-gray-400 hover:text-white hover:bg-gray-700 focus:outline-none focus:ring-2 focus:ring-inset focus:ring-white"> <span className="sr-only">Open main menu</span> {open ? ( <XIcon className="block h-6 w-6" aria-hidden="true" /> ) : ( <MenuIcon className="block h-6 w-6" aria-hidden="true" /> )} </Disclosure.Button> </div> <div className="flex-1 flex items-center justify-center sm:items-stretch sm:justify-start"> <div className="flex-shrink-0 flex items-center"> <img className="block lg:hidden h-8 w-auto" src="https://tailwindui.com/img/logos/workflow-mark-indigo-500.svg" alt="Workflow" /> <img className="hidden lg:block h-8 w-auto" src="https://tailwindui.com/img/logos/workflow-logo-indigo-500-mark-white-text.svg" alt="Workflow" /> </div> <div className="hidden sm:block sm:ml-6"> <div className="flex space-x-4"> {navigation.map((item) => ( <a key={item.name} href={item.href} className={classNames( item.current ? "bg-gray-900 text-white" : "text-gray-300 hover:bg-gray-700 hover:text-white", "px-3 py-2 rounded-md text-sm font-medium" )} aria-current={item.current ? "page" : undefined} > {item.name} </a> ))} </div> </div> </div> <div className="absolute inset-y-0 right-0 flex items-center pr-2 sm:static sm:inset-auto sm:ml-6 sm:pr-0"> <button type="button" className="bg-gray-800 p-1 rounded-full text-gray-400 hover:text-white focus:outline-none focus:ring-2 focus:ring-offset-2 focus:ring-offset-gray-800 focus:ring-white" > <span className="sr-only">View notifications</span> <BellIcon className="h-6 w-6" aria-hidden="true" /> </button> {/* Profile dropdown */} <Menu as="div" className="ml-3 relative"> <div> <Menu.Button className="bg-gray-800 flex text-sm rounded-full focus:outline-none focus:ring-2 focus:ring-offset-2 focus:ring-offset-gray-800 focus:ring-white"> <span className="sr-only">Open user menu</span> <img className="h-8 w-8 rounded-full" src="https://images.unsplash.com/photo-1472099645785-5658abf4ff4e?ixlib=rb-1.2.1&ixid=eyJhcHBfaWQiOjEyMDd9&auto=format&fit=facearea&facepad=2&w=256&h=256&q=80" alt="" /> </Menu.Button> </div> <Transition as={Fragment} enter="transition ease-out duration-100" enterFrom="transform opacity-0 scale-95" enterTo="transform opacity-100 scale-100" leave="transition ease-in duration-75" leaveFrom="transform opacity-100 scale-100" leaveTo="transform opacity-0 scale-95" > <Menu.Items className="origin-top-right absolute right-0 mt-2 w-48 rounded-md shadow-lg py-1 bg-white ring-1 ring-black ring-opacity-5 focus:outline-none"> <Menu.Item> {({ active }) => ( <a href="#" className={classNames( active ? "bg-gray-100" : "", "block px-4 py-2 text-sm text-gray-700" )} > Your Profile </a> )} </Menu.Item> <Menu.Item> {({ active }) => ( <a href="#" className={classNames( active ? "bg-gray-100" : "", "block px-4 py-2 text-sm text-gray-700" )} > Settings </a> )} </Menu.Item> <Menu.Item> {({ active }) => ( <a href="#" className={classNames( active ? "bg-gray-100" : "", "block px-4 py-2 text-sm text-gray-700" )} > Sign out </a> )} </Menu.Item> </Menu.Items> </Transition> </Menu> </div> </div> </div> <Disclosure.Panel className="sm:hidden"> <div className="px-2 pt-2 pb-3 space-y-1"> {navigation.map((item) => ( <Disclosure.Button key={item.name} as="a" href={item.href} className={classNames( item.current ? "bg-gray-900 text-white" : "text-gray-300 hover:bg-gray-700 hover:text-white", "block px-3 py-2 rounded-md text-base font-medium" )} aria-current={item.current ? "page" : undefined} > {item.name} </Disclosure.Button> ))} </div> </Disclosure.Panel> </> )} </Disclosure> ); }
- Go to your React application and create a
src/Navigation
folder. - Create a
src/Navigation/Navbar.js
file in thesrc/Navigation
directory. - Paste the Tailwind CSS boilerplate navigation menu and the navigation menu you copied over before in your file. Look for other Tailwind imports at the top of the file and install their packages accordingly. The function name may be something generic, like
Example
, but rename it to something more specific, likeNavbar
. - Go to your
App.js
and importsrc/Navigation/Navbar.js
.
Be sure to also import index.css
since it contains the configuration for Tailwind CSS:
1 2 3 4 5 6 7 8 9 10 11
import "./index.css"; import Navbar from "./Navigation/Navbar.js"; function App() { return; <div> <Navbar /> </div>; } export default App;
- Run
npm start
. Your navbar should look like this:
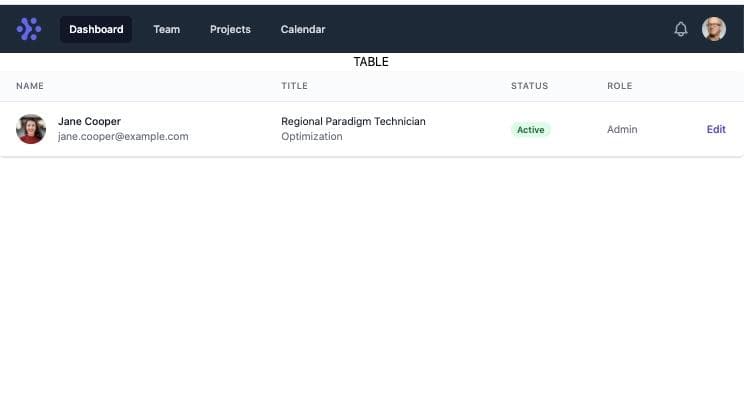
- Check the Tailwind CSS docs if you want to customize it further. You can add elements into a CSS class in-line to your tag’s
className
or, for example, add colors to yourtailwind.config.js
file.
You've now seen two easy ways to create a navbar in your React app. Bootstrap and Tailwind CSS are both excellent choices for quickly styling an application while allowing for more personalization.
The free components are great for quick-start applications, but be sure to check each framework's documentation and libraries to see how you can use props effectively to enhance the functionality and appearance of your navbar contents
If you’re looking to ship your project faster or if you are building on a larger scale, you might want to check us (Retool) out. We provide a complete set of building blocks straight out of the box. You can assemble an app in minutes by dragging and dropping from Retool's pre-built components and quickly connect to backend databases and APIs. We think that Retool is great for building internal tools, admin panels, and CRUD interfaces so you can quickly automate the manual and tedious tasks saving you time for those more bespoke React projects. For more info, check out the docs.
Reader